
CBSE Guess > Papers > Important Questions > Class XII > 2013 > Computer Science > Computer Science - by Mr. Sandip Kumar Srivastava
CBSE CLASS XII
Computer Science (C++ and Data Structure)
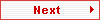
- class Test
{ char paper[12];
int marks;
};
Rewrite the above class definition, by defining a default constructor that assign
“computer” to paper and marks to 100.
- Define a class BALANCED_MEAL in C++ with following description:
Private Members:
Access_no |
Integer |
Food_Name |
String of 25 characters |
Calories |
Integer |
Food_type |
String |
Cost |
Float |
AssignAccess( ) Generates random numbers between 10 and 99 and return it.
Public Members
- A function INTAKE( ) to allow the user to enter the values of Food_Name,
Calories, Food_type, cost and call function AssignAccess( ) to assign Access_no.
- A function OUTPUT( ) to allow user to view the content of all the data members, if the Food_type is Fruit.Otherwise display message, “Display only for fruit”
Write a function that reads binary file CONSUMER.DAT containing records of above class, and copy those records to CONSUMERAREA.DAT , whose area is same as given by user. Also, display the number of such records found.
- Differentiate between an identifier and keywords.
- Name the header files, to which following inbuilt function belong to:
- log10( )
- arc( )
- What is Function Overloading? Give an example in C++ to illustrate the same.
- In Object Oriented Programming, which concept is illustrated by Function1 and Function2 together? Write the statement to call these functions.
- What is the scope of two data members of the class AirIndia? What does the scope of data members depend upon?
- Define a class cricket in C++ with the following description:
Private members:
- Target_score of type integer
- Overs_bowled of type integer
- Extra_time of type integer
- Penalty of type integer
- Cal_penalty() a member function to calculate penalty as follows:
If extra_time<=10, penalty=1
If extra_time>10 but <=20 penalty=2, otherwise, penalty=5
Public members:
- A fuinction Extradata () to allow user to enter values for target_score, over_bowled, Extra_time.
- A function DspData( ) to allow user to view the contents of all data members.
- Write a function in C++ which accepts a 2D array of integers and its size as arguments and display the elements which lie on diagonals.
[Assuming the 2D array to be a square matrix with odd dimension, i.e., 3x3, 5x5, etc..]
Example, if the array contents is
5 4 3
6 7 8
1 2 9
Output through the function should be :
Diagonal One : 5 7 9
Diagonal Two: 3 7 1
- An array Arr[15][20] is stored in the memory along the row with each element occupying 4 bytes. Find out the Base Address and address of the element Arr[3][2], if the element [5][2] is stored at the address 1500.
- Give the necessary declaration of queue containing integer. Write a user defined function in C++ to delete an integer from the queue. The queue is to be implemented as a linked list
- Write a function in C++ to print the sum of all the values which are either divisible by 2 or are divisible by 3 present in a two-dimensional array passed as the argument to the function.
- Evaluate the following postfix notation of expression:
- Write a function in C++ to count the number of alphabets present in a textfile “Para.Txt”.
- Write a function in C++ to add new objects at the bottom of a binary file “Student.Dat”, assuming the binary file is containing the object of the following class
class STUD
{
int Rno;
char Name[20];
public:
void Enter ()
{
cin>>Rno;
gets(Name);
}
void Display()
{
cout<<Rno<<Name<<endl;
}};
- What do you mean by static variable? Explain with help of example.
- Write the header files to which the following functions belong:
- getc ( )
- isalnum ( )
- scanf ( )
- getxy ( )
- How member functions and non member functions differ?
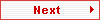
Submitted By : Mr. Sandip Kumar Srivastava
Email : [email protected] |
|