
CBSE Guess > Papers > Important Questions > Class XII > 2010 > Computer Science > Computer Science By Ravi Kiran
CBSE CLASS XII
OUTSIDE DELHI : 2005
Q. 4.a. Observe the program segment given below carefully , and answer the question that follows :
class Member
{ int Member_no ;
char Member_name[20] ;
public :
//function to enter Member details
void enterdetails ( ) ;
//function to display Member details
void showdetails ( ) ;
//function to return Member_no
int RMember_no( ) {return Member_no ;}
} ;
void Update (Member NEW)
{ fstream File ;
File.open(“MEMBER.DAT” , ios :: binary l ios :: in l ios :: out) ;
Member OM ;
int Recordsread = 0, Found = 0 ;
while (!Found && File.read((char*) & OM, sizeof(OM)))
{ Recordsread++ ;
if (NEW.RMember_no( ) == OM.RMember_no( ))
{ _____________ //Missing Statement
File.write((char*) & NEW , sizeof(NEW) ;
Found = 1 ;
}
else
File.write((char*) & OM, sizeof(OM)) ;
}
if (!Found)
cout<<”Record for modification does not exist” ;
File.close( ) ;
}
If the function Update( ) is supposed to modify a record in file MEMBER.DAT with the values of
Member NEW passed to its argument, write the appropriate statement for Missing statement using
seekp( ) or seekg( ), whichever needed, in the above code that would write the modified record at its
proper place.
Q. 4.b. Write a function in C++ to count and display the number of lines not starting with alphabet ‘A’
present in a text file “STORY.TXT”.
Example :
If the file “STORY.TXT” contains the following lines,
The rose is red.
A girl is playing there.
There is a playground.
An aeroplane is in the sky.
Numbers are not allowed in the password.
The function should display the output as 3
Q. 4.c. Given a binary file APPLY.DAT, containing records of the following class Applicant type 3
class Applicant
{ char A_Rno[10] ; //Roll number of applicant
char A_Name[30] ; //Name of applicant
int A_Score ; //Score of applicant
public :
void Enrol( )
{ gets(A_Rno) ; gets(A_Name) ; cin >> A_Score ;
}
void Status( )
{
cout << setw(12) << A_Admno ;
cout << setw(32) << A_Name ;
cout << setw(3) << A_Score << endl ;
}
int ReturnScore( ) {return A_Score ;}
} ;
Write a function in C++, that would read contents of file APPLY.DAT and display the details of
those Students whose A_Score is above 70.
DELHI : 2004
Q. 4.a. Assuming that a text file named FIRST.TXT contains some text written into it, write a function
named vowelwords( ), that reads the file FIRST.TXT and creates a new file named SECOND.TXT, to
contain only those words from the file FIRST.TXT which start with start with a lowercase vowel (i.e.
with ‘a’, ’e’, ’I’, ‘o’, ‘u’). For example if the file FIRST.TXT contains
Carry umbrella and overcoat when it rains
Then the file SECOND.TXT shall contain:
umbrella and overcoat it
Q. 4b. Assuming the class Computer as follows:
class computer
{ char chiptype[10];
int speed;
public:
void getdetails( )
{ get(chiptype);
cin>>speed;
}
void showdetails( )
{ cout<<”Chip”<<chiptype<”Speed = “speed;
}
} ;
Q. 4c. Write a function readfile( ) to read all the records present in already existing binary file
SHIP.DAT and display them on the screen, also count the number of records present in the file.
DELHI : 2003
Q. 4.a. Write a user defined function in C++ to read the content from a text file NOTES.TXT, count and
display the number of blank spaces present in it.
Q. 4.b. Assuming a binary file FUN.DAT is containing objects belonging to a class LAUGHTER (as
defined below).Write a user defined function in C++ to add more objects belonging to class
LAUGHTER at the bottom of it.
class LAUGHTER
{ int Idno;// Identification number
char Type[5]; //LAUGHTER Type
char Desc[255]; //Description
public :
void Newentry( )
{ cin>>Idno;gets(Type);gets(Desc);}
void Showonscreen( )
{ cout<<Idno<<”:”<<Type<<endl<<Desc<<endl;}
DELHI : 2002
Q. 4.a. What is the difference between pub( ) and write ( )?
Q. 4.b. Write a C++ program, which initializes a string variable to the content “Time is a great teacher
but unfortunately it kills all its pupils. Berlioz” and outputs the string one character at a time to the
disk file OUT.TXT.You have to include all the header files if required.
DELHI : 2001
Q. 4.a. Distinguish between ios::out and ios::app.
The ios::out mode opens the file in output mode only.
The ios::app mode opens the file in append mode, where the file can be appended.
Q. 4.b. Consider the class declaration
class FLIGHT
{ protected:
int flight_no;
char destination[20];
float distance;
public:
void INPUT( ); //To read an object from the keyboard
void write_file(int); //To write N objects into the file,
//Where N is passed as argument.
void OUTPUT( ); //To display the file contents on the monitor.
};
Complete the member functions definitions.
2000
Q. 4.a. Name two member functions of ofstream class.
Q. 4.b. Assuming the class DRINKS defined below, write functions in C++ to perform the following:
(i)Write the objects of DRINKS to a binary file.
(ii) Read the objects of DRINKS from binary file and display them on screen when DNAME
has
value "INDY COLA".
class DRINKS
{
int DCODE;
char DNAME[13]; //Name of the drink
int DSIZE; //Size in liters float DPRICE;public:
void getdrinks( ) {cin>>DCODE>>DNAME>>DSIZE>>DPRICE;}
void showdrinks( )
{cout<<DCODE<<DNAME<<DSIZE<<DPRICE<<endl;}
char *getname( ){return DNAME;}
};
1999
Q. 4a. Differentiate between functions read() and write().
Q. 4b. Assuming the class FLOPPYBOX, write a function in C++ to perform following:
(i) Write the objects of FLOPPYBOX to a binary file.
(ii) Reads the objects of FLOPPYBOX from binary file and display them on screen.
class FLOPPYBOX
{ int size;
char name[10];
public:
void getdata(){cin>>size;gets(name);}
void showdata(){cout<<size<<" "<<name<<endl;}
};
1998
Q. 4.a. Write name of two member functions belonging to fstream class.
Q. 4. b. Assuming the class EMPLOYEE given below, write functions in C++ to perform the
following:
(i) Write the objects of EMPLOYEE to a binary file.
(ii) Read the objects of EMPLOYEE from binary file and display them on the screen.
class EMPLOYEE
{
int ENO;
char ENAME[10];
public:
void GETIT( )
{
cin>>ENO;
gets(ENAME);
}
void SHOWIT( )
{
cout< < ENO<<ENAME<<endl;
}
};
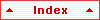
Paper By Mr. Ravi Kiran
Email Id : mrkdata@yahoo.com |