
CBSE Guess > Papers > Important Questions > Class XII > 2010 > Computer Science > Computer Science By Ravi Kiran
CBSE CLASS XII
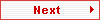
(e) Find the output of the following program : 2
#include<iostream.h>
#include<string.h>
#include<ctype.h>
void Change(char Msg[],int Len)
{
for (int Count =0; Count<Len; Count++ )
{
if (islower(Msg[Count]))
Msg[Count]= toupper{Msg[Count]);
else if (isupper(Msg[Count]))
Msg[Count]= tolower(Msg[Count]);
else if (isdigit(Msg[Count]))
Msg[Count]= Msg[Count] + 1;
else Msg[Count] = ‘*’ ;
}
}
void main()
{
char Message[] = "2005 Tests ahead";
int Size = strlen(Message);
Change(Message,Size);
cout<<Message<<endl;
for (int C = 0,R=Size-l;C<=Size/2; C++,R--)
{
char Temp = Message[C];
Message[C]= Message[R];
Message[R]= Temp;
}
cout<<Message<<endl;
}
(f) Observe the following program GAME.CPP carefully, if the value of Num entered by
the user is 14, choose the correct possible output(s) from the options from (i) to (iv),
and justify your option. 2
//Program : GAME.CPP
#include<stdlib.h>
#include<iostream.h>
void main()
{
randomize();
int Num, Rndnum;
cin>>Num;
Rndnum = random(Num) + 7;
for (int N = 1; N<=Rndnum ; N++)
cout<<N<<" ";
}
Output Options :
(i) 1 2 3
(ii) 1 2 3 4 5 6 7 8 9 10 11
(iii) 1 2 3 4 5
(iv) 1 2 3 4
2. (a) Define the term Data Encapsulation in the context of Object Oriented Programming.
Give a suitable example using a C++ code to illustrate the same. 2
(b) Answer the questions (i) and (ii) after going through the following class : 2
class Exam
{
int Marks;
char Subject[20];
public:
Exam() //Function 1
{
Marks = 0;
strcpy (Subject,”Computer”);
}
Exam(char S[]) //Function 2
{
Marks = 0;
strcpy(Subject,S);
}
Exam(int M) //Function 3
{
Marks = M;
strcpy(Subject,”Computer”);
}
Exam(char S[], int M) //Function 4
{
Marks = M;
strcpy(Subject,S);
}
};
(i) Write statements in C++ that would execute Function 3 and Function 4 of
class Exam.
(ii) Which feature of Object Oriented Programming is demonstrated using
Function 1, Function 2, Function 3 and Function 4 in the above class Exam ?
(c) Define a class Travel in C++ with the following descriptions : 4
Private Members :
TravelCode of type long
Place of type character array (string)
No_of_travellers of type integer
No_of_buses of type integer
Public Members :
A constructor to assign initial values of TravelCode as 201, Place as “Nainital”,
No_of_travellers as 10, No_of_buses as
A function NewTravel() which allows user to enter TravelCode, Place and
No_of_travellers. Also, assign the value of No_of_buses as per the following
conditions :
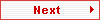
Paper By Mr. Ravi Kiran
Email Id : [email protected] |