
CBSE Guess > Papers > Important Questions > Class XII > 2011 > Computer Science > Computer Science By Deepa
CBSE CLASS XII
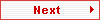
Arrays (Questions with Answer)
Page 1 of 3
- Define a function shift() to shift all odd elements towards left and even to right without changing order of numbers.
Example: If input: 1,5,7,8,9,2,10
Output: 1,5,7,9,8,2,10.
Ans:
void shift(int a[10],int n)
{
int b[10],i,k=0;
for(i=0;i<n;i++)
{
if(a[i]%2= =1)
{
b[k]=a[i];
k++;
}
}
for(i=0;i<n;i++)
{ if(a[i]%2= =0)
{ b[k]=a[i];
k++;
}
}
for(i=0;i<n;i++)
cout<<b[i]<<" ";
}
- Given two arrays A and B. Array ‘A’ contains all the elements of ‘B’ but one more element extra. Write a c++ function which accepts array A and B and its size as arguments/ parameters and find out the extra element in Array A. (Restriction: array elements are not in order)
Example : -
If Array A is {14, 21, 5, 19, 8, 4, 23, 11}
and Array B is {23, 8, 19, 4, 14, 11, 5 }
Then output will be 21 (extra element in Array A)
Ans:
void extra(int a[10],int m,int b[10],int n)
{
int i,j,flag;
for(i=0;i<m;i++)
{ flag=0;
for(j=0;j<n;j++)
if(a[i]==b[j])
{ flag=1;
break;
}
if(flag==0)
cout<<a[i]<<" is the extra element\n";
}
}
- Write C++ function to Arrange(int [],int) to arrange all the negative and positive numbers from left to right.
Example : -
If an array of 10 elements initially contains { 4,5,6,-7,8,-2,-10,1,13,-20} . Then the function rearrange them in following manner { -20,-10,-7,-2 1,4,5,6,8,13}
Ans:
void arrange(int a[10],int n)
{
int i,j,temp;
for(i=0;i<n;i++)
{ for(j=0;j<n-1;j++)
if(a[j]>a[j+1])
{ temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
}
for(i=0;i<n;i++)
cout<<a[i]<<" ";
}
- Write a user defined function in C++ to find and display the row sums of a two dimensional array.
Ans:
void rowsum(int a[10][10],int m,int n)
{
int i,j,rsum;
for(i=0;i<m;i++)
{ rsum=0;
for(j=0;j<n;j++)
rsum+=a[i][j];
cout<<"\nSum of row "<<i+1<<" is -"<<rsum;
}
}
- Write a user defined function in C++ to find and display the column sums of a two dimensional array.
Ans:
void columnsum(int a[10][10],int m,int n)
{
int i,j,csum;
for(i=0;i<n;i++)
{ csum=0;
for(j=0;j<m;j++)
csum+=a[j][i];
cout<<"\nSum of column "<<i+1<<" is ->"<<csum;
}
}
- Write a function in C++ to print the product of each row of a two dimensional array passed as the arguments of the function
Ans:
void rowproduct(int a[10][10],int m,int n)
{
int i,j,rp;
for(i=0;i<m;i++)
{ rp=1;
for(j=0;j<n;j++)
rp*=a[j][i];
cout<<"\nProduct of row "<<i+1<<" is ->"<<rp;
}
}
- Write a function in C++ which accepts a 2D array of integers and its size as arguments and displays the elements which lie on diagonals. [Assuming the 2D Array to be a square matrix with odd dimension i.e. 3×3, 5×5, 7×7 etc.]
Example, if the array content is
5 4 3
6 7 8
1 2 9
Output through the function should be:
Diagonal 1 : 5 7 9 Diagonal 2 : 3 7 1
Ans:
void diagonal(int a[10][10],int m,int n)
{
int i,j;
cout<<"\nDiagonal 1:";
for(i=0;i<m;i++)
{ for(j=0;j<n;j++)
if(i==j)
cout<<a[i][j]<<" ";
}
cout<<"\n\nDiagonal 2:";
for(i=0;i<m;i++)
{ for(j=0;j<n;j++)
if(i+j==n-1)
cout<<a[i][j]<<" ";}
}
- Write a user defined function in C++ which accepts a squared integer matrix with odd dimensions (3*3, 5*5 …) & display the square of the elements which lie on both diagonals. For ex. :
2 5 7
3 7 2
5 6 9
The output should be :
Diagonal one : 4, 49, 81
Diagonal two : 49, 49, 25
Ans:
void diagonalsquare(int a[10][10],int m,int n)
{
int i,j;
cout<<"\nDiagonal one :";
for(i=0;i<m;i++)
{ for(j=0;j<n;j++)
if(i==j)
cout<<a[i][j]*a[i][j]<<",";}
cout<<"\n\nDiagonal two:";
for(i=0;i<m;i++)
{ for(j=0;j<n;j++)
if(i+j==n-1)
cout<<a[i][j]*a[i][j]<<",";
}
}
- Write a user defined function in C++ which accepts a squared integer matrix with odd dimensions (3*3, 5*5..) & display the sum of the middle row & middle column elements. For ex. :
2 5 7
3 7 2
5 6 9
The output should be :
Sum of middle row = 12
Sum of middle column = 18
Ans:
void middlesum(int a[10][10],int m,int n)
{
int i,j,mrsum=0,mcsum=0;
for(i=0;i<n;i++)
mrsum+=a[m/2][i];
cout<<"\nSum of middle row="<<mrsum;
for(i=0;i<m;i++)
mcsum+=a[i][n/2];
cout<<"\nSum of middle column="<<mcsum;
}
- Write a user-defined function named Lower_half() which takes 2D array A, with size N rows and N columns as argument and prints the lower half of the array.
Eg.
Input
2 3 1 5 0
7 1 5 3 1
2 5 7 8 1
0 1 5 0 1
3 4 9 1 5
the output will be
2
7 1
2 5 7
0 1 5 0
3 4 9 1 5
Ans:
void lower_half(int a[10][10],int n)
{
int i,j;
for(i=0;i<n;i++)
{ cout<<endl;
for(j=0;j<n;j++)
if(i>=j)
cout<<a[i][j]<<" ";
}
}
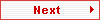
Submitted By Deepa
Email Id : [email protected]
About Author: Computer Teacher From Sunrise English Pvt. School |
|